Web Page Images and Thumbnails
Preamble
Many applications would be improved by displaying thumbnails of web pages.
In a previous article (on my, now abandoned, dotnetjunkies blog) I showed some sample code of how to do this with the WebBrowser control using Framework 1.1. Unfortunately, many readers seemed to encounter problems using these samples, compounded by breaking changes in Framework 2.0.
In light of this, I have reworked the article and have created a working solution to demonstrate the code, available for download.
Visual Studio Solution
Download Visual Studio 2005 Solution (C#) : Zipped (???Kb)
Research
I started off by Googling.
C# web page thumbnail, C# web site thumbnail, and others give plenty of links about how to make "websites of thumbnails" rather than "thumbnails of websites" which wasn't what I wanted.
Along the way, I found Display Web Page ThumbShots without Hosting Images which discusses two sites in particular (Thumbshots.org and Alexa) which provide web capabilites for thumbnails by serving up images. For more information, see Alexa Developer's Corner - Thumbnail Images.
If you want to write a web application, these services may be very useful for you. They might also be useful if you want to to write certain types of windows applications (for example, smart client apps on PocketPC where storage space is at a premium). However, they really don't meet my needs because I want to generate the images myself.
My next thought was to see if I could use Internet Explorer to get the images I needed. IE is provided as a component by the WebBrowser control.
Firefox
The code samples shown do not use Firefox.
However, if you are just looking for a quick & simple way of getting an image of an entire web page, I highly recommend the Firefox Add-On Save As Image.
The Code
Once the WebBrowser control is added to a form, the following code can be used to obtain an image of the displayed web page from the control.
Please note that the following code will only obtain an image of a portion of the web page. I am researching how it might be possible to obtain the complete page, but I haven't found a way yet.
This code captures the displayed page when the DocumentCompleted event fires:
Once we have the image, it is then a simple matter create and save a thumbnail:
Here is a thumbnail of the MSN homepage, generated by this sample:
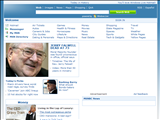
Many applications would be improved by displaying thumbnails of web pages.
In a previous article (on my, now abandoned, dotnetjunkies blog) I showed some sample code of how to do this with the WebBrowser control using Framework 1.1. Unfortunately, many readers seemed to encounter problems using these samples, compounded by breaking changes in Framework 2.0.
In light of this, I have reworked the article and have created a working solution to demonstrate the code, available for download.
Visual Studio Solution
Download Visual Studio 2005 Solution (C#) : Zipped (???Kb)
Research
I started off by Googling.
C# web page thumbnail, C# web site thumbnail, and others give plenty of links about how to make "websites of thumbnails" rather than "thumbnails of websites" which wasn't what I wanted.
Along the way, I found Display Web Page ThumbShots without Hosting Images which discusses two sites in particular (Thumbshots.org and Alexa) which provide web capabilites for thumbnails by serving up images. For more information, see Alexa Developer's Corner - Thumbnail Images.
If you want to write a web application, these services may be very useful for you. They might also be useful if you want to to write certain types of windows applications (for example, smart client apps on PocketPC where storage space is at a premium). However, they really don't meet my needs because I want to generate the images myself.
My next thought was to see if I could use Internet Explorer to get the images I needed. IE is provided as a component by the WebBrowser control.
Firefox
The code samples shown do not use Firefox.
However, if you are just looking for a quick & simple way of getting an image of an entire web page, I highly recommend the Firefox Add-On Save As Image.
The Code
Once the WebBrowser control is added to a form, the following code can be used to obtain an image of the displayed web page from the control.
Please note that the following code will only obtain an image of a portion of the web page. I am researching how it might be possible to obtain the complete page, but I haven't found a way yet.
This code captures the displayed page when the DocumentCompleted event fires:
+展开
-C#
private void OnDocumentCompleted(object sender, WebBrowserDocumentCompletedEventArgs e)
{
WebBrowser browser = (sender as WebBrowser);
if (browser != null)
{
mshtml.IHTMLDocument2 document = (browser.Document.DomDocument as mshtml.IHTMLDocument2);
if (document != null)
{
mshtml.IHTMLElement element = (document.body as mshtml.IHTMLElement);
if (element != null)
{
IHTMLElementRender render = (element as IHTMLElementRender);
if (render != null)
{
using (Graphics graphics = this.CreateGraphics())
{
IntPtr hdcDestination = graphics.GetHdc();
render.DrawToDC(hdcDestination);
IntPtr hdcMemory = GDI32.CreateCompatibleDC(hdcDestination);
IntPtr bitmap = GDI32.CreateCompatibleBitmap(
hdcDestination,
browser.ClientRectangle.Width,
browser.ClientRectangle.Height
);
if (bitmap != IntPtr.Zero)
{
IntPtr hOld = (IntPtr)GDI32.SelectObject(hdcMemory, bitmap);
GDI32.BitBlt(
hdcMemory,
0, 0,
browser.ClientRectangle.Width, browser.ClientRectangle.Height,
hdcDestination,
0, 0,
TernaryRasterOperations.SRCCOPY
);
GDI32.SelectObject(hdcMemory, hOld);
GDI32.DeleteDC(hdcMemory);
graphics.ReleaseHdc(hdcDestination);
SaveThumbnail(Image.FromHbitmap(bitmap));
}
}
}
}
}
}
}
{
WebBrowser browser = (sender as WebBrowser);
if (browser != null)
{
mshtml.IHTMLDocument2 document = (browser.Document.DomDocument as mshtml.IHTMLDocument2);
if (document != null)
{
mshtml.IHTMLElement element = (document.body as mshtml.IHTMLElement);
if (element != null)
{
IHTMLElementRender render = (element as IHTMLElementRender);
if (render != null)
{
using (Graphics graphics = this.CreateGraphics())
{
IntPtr hdcDestination = graphics.GetHdc();
render.DrawToDC(hdcDestination);
IntPtr hdcMemory = GDI32.CreateCompatibleDC(hdcDestination);
IntPtr bitmap = GDI32.CreateCompatibleBitmap(
hdcDestination,
browser.ClientRectangle.Width,
browser.ClientRectangle.Height
);
if (bitmap != IntPtr.Zero)
{
IntPtr hOld = (IntPtr)GDI32.SelectObject(hdcMemory, bitmap);
GDI32.BitBlt(
hdcMemory,
0, 0,
browser.ClientRectangle.Width, browser.ClientRectangle.Height,
hdcDestination,
0, 0,
TernaryRasterOperations.SRCCOPY
);
GDI32.SelectObject(hdcMemory, hOld);
GDI32.DeleteDC(hdcMemory);
graphics.ReleaseHdc(hdcDestination);
SaveThumbnail(Image.FromHbitmap(bitmap));
}
}
}
}
}
}
}
Once we have the image, it is then a simple matter create and save a thumbnail:
+展开
-C#
private void SaveThumbnail(Image image)
{
if (image != null)
{
Bitmap thumbnail = new Bitmap(160, 120, PixelFormat.Format24bppRgb);
thumbnail.SetResolution(image.HorizontalResolution, image.VerticalResolution);
using (Graphics resize = Graphics.FromImage(thumbnail))
{
resize.InterpolationMode = InterpolationMode.HighQualityBicubic;
resize.DrawImage(image,
new Rectangle(0, 0, 160, 120),
new Rectangle(0, 0, _webBrowser.ClientRectangle.Width, _webBrowser.ClientRectangle.Height),
GraphicsUnit.Pixel);
}
thumbnail.Save(_file.FullName, ImageFormat.Png);
}
}
{
if (image != null)
{
Bitmap thumbnail = new Bitmap(160, 120, PixelFormat.Format24bppRgb);
thumbnail.SetResolution(image.HorizontalResolution, image.VerticalResolution);
using (Graphics resize = Graphics.FromImage(thumbnail))
{
resize.InterpolationMode = InterpolationMode.HighQualityBicubic;
resize.DrawImage(image,
new Rectangle(0, 0, 160, 120),
new Rectangle(0, 0, _webBrowser.ClientRectangle.Width, _webBrowser.ClientRectangle.Height),
GraphicsUnit.Pixel);
}
thumbnail.Save(_file.FullName, ImageFormat.Png);
}
}
Here is a thumbnail of the MSN homepage, generated by this sample:
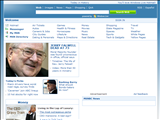
加支付宝好友偷能量挖...